How to use the OpenAI Evals API
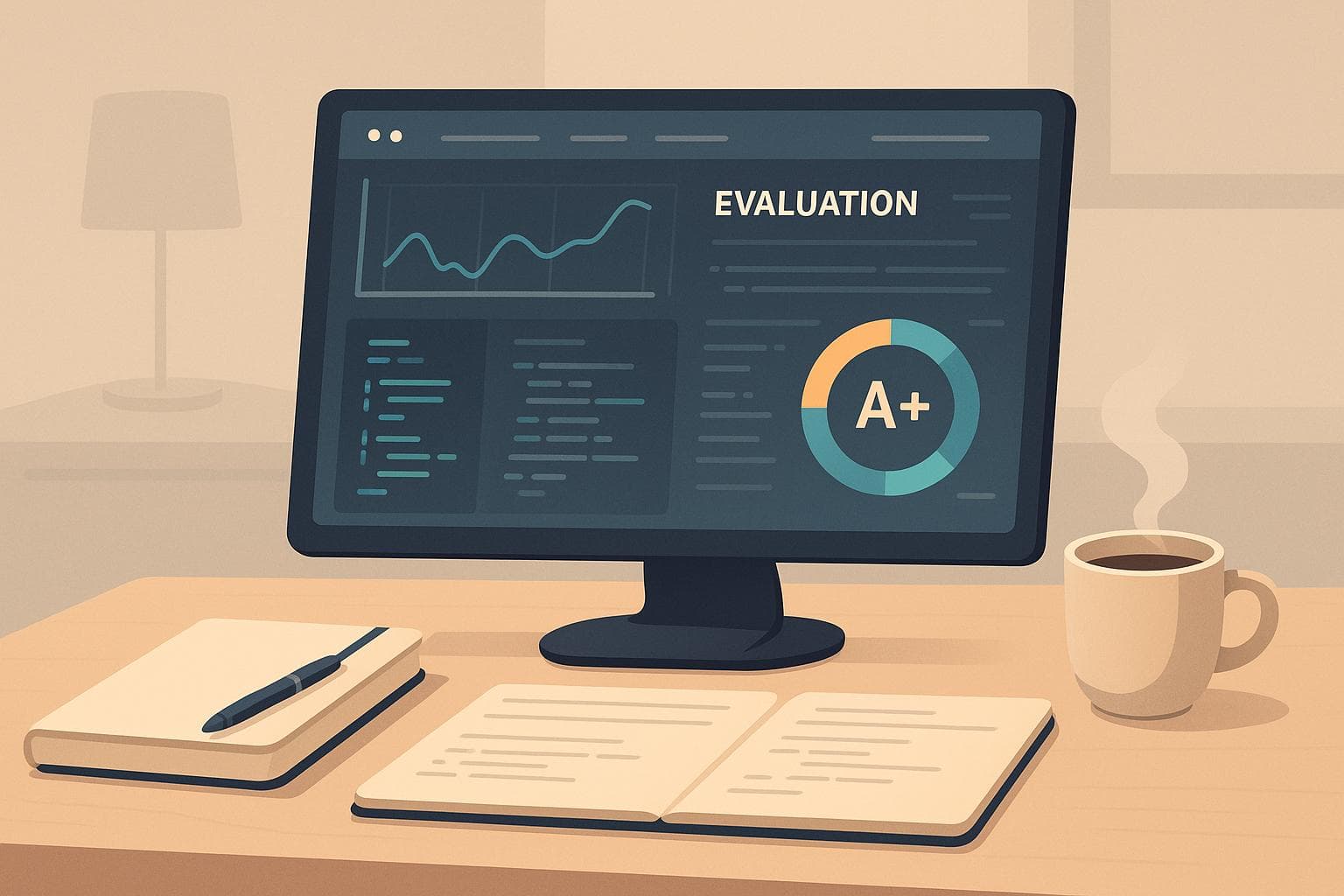
How to use the OpenAI Evals API
The OpenAI Evals API helps you test and improve AI models by automating quality checks, saving time, and ensuring consistent performance. It’s perfect for developers and businesses who rely on language models for tasks like chatbots, content creation, or compliance. Here's what you can do with it:
- Automate evaluations: Test up to 500 responses at once, saving 20+ hours weekly.
- Track performance: Compare model versions side by side and monitor quality over time.
- Ensure compliance: Align outputs with business or regulatory standards, like including required disclosures.
For example, a SaaS company improved chatbot resolution rates from 68% to 89% in just three weeks using this API. Another firm reduced response latency by 30% while ensuring compliance with U.S. standards.
Quick Start:
- Set up your environment: Requires Python 3.9+,
openai
library, and Git LFS. - Define your tests: Use evaluation types like classification, fact-checking, or safety.
- Run and refine: Automate tests, analyze results, and adjust prompts for better outcomes.
Whether you're building customer-facing tools or internal applications, the Evals API ensures your AI is accurate, fast, and reliable. Keep reading to learn how to set it up and maximize its potential.
Getting Started with OpenAI Evals API
Required Credentials
To use the OpenAI Evals API, you'll need an active OpenAI account and a valid API key. This key acts as your authentication token, so make sure to keep it secure. For enterprise setups, rely on secure secret management practices.
Initial Setup Steps
To work with the Evals API, you need to set up a few technical components. Here’s what you’ll need:
Component | Version/Requirement | Purpose |
---|---|---|
Python | 3.9 or higher | Core runtime environment |
openai | ≥1.20.0 | Evals API support |
git-lfs | ≥3.0 | Data versioning |
pydantic | ≥2.5 | Schema validation |
Follow these steps to get started:
- Install required packages: Run
pip install openai git-lfs pydantic
- Configure Git LFS: Use
git lfs install && git lfs pull
- Set API key: Add your key to environment variables as
OPENAI_API_KEY=sk-...
For production environments, consider adding IP whitelisting through the OpenAI dashboard. Enterprise users should also set up quarterly key rotations and secure secret management systems. This setup ensures smooth integration with various programming environments.
Compatible Technologies
Python is the primary language for the Evals API, offering native support through the openai
library. However, the REST API allows you to integrate with other programming languages. Here’s a quick look at supported integrations:
Language/Framework | Integration Method | Use Case |
---|---|---|
JavaScript/Node.js | axios or fetch | Web applications |
Java | Apache HttpClient | Enterprise systems |
C# | RestSharp | .NET applications |
For those using Microsoft's ecosystem, the Azure OpenAI Service provides a similar Evaluation API with unique configuration options. It includes features like Azure Monitor integration and compliance certifications.
To make sure your setup is working, run the command openai.evals.list(limit=1)
to test API connectivity. For CI/CD pipelines, secure your API credentials by using protected variables.
3 Main Steps of the Evals API
Step 1: Define Your Evaluation
Start by setting up your evaluation using an EvalSpec
object. This outlines the test criteria and success metrics you'll use.
eval_spec = {
"model_specs": [{"model": "gpt-4"}],
"eval_type": "modelgraded/classification",
"input_prompts": ["Translate 'Hello' to Spanish"],
"ideal_responses": ["Hola"],
"output_requirements": ["no_code"]
}
Here’s a quick breakdown of evaluation types and their applications:
Evaluation Type | Use Case | Example Criteria |
---|---|---|
Classification | Organizing content | Sentiment analysis, topic detection |
Fact-checking | Verifying accuracy | Knowledge base validation |
Safety | Moderating content | Harmful content detection |
Style | Ensuring writing standards | Brand voice compliance |
Once your evaluation is ready, the next step is running the tests.
Step 2: Run the Tests
A great example of systematic testing comes from Shopify. In early 2024, their team continuously evaluated product description generators to improve performance.
To execute your evaluation, use the following code:
from openai import Evals
eval_run = Evals.Run.create(
eval_spec_id="eval_123",
test_data={"prompts": ["Explain quantum computing"]},
eval_settings={"temperature": 0.7}
)
For larger datasets, you can streamline the process:
- Use
async=True
for non-blocking execution. - Divide datasets into chunks of 500 entries.
- Track progress through the results dashboard.
After running the tests, you’ll need to analyze the results and make improvements.
Step 3: Analyze and Refine
Review the results to identify areas for improvement. For example, The New York Times tech team boosted summary quality by 32% by combining automated metrics with human scoring.
Key metrics to monitor include:
Metric | Target | Action if Below Target |
---|---|---|
Pass Rate | >95% | Adjust prompt engineering |
Response Time | P90 < 2s | Shorten input length |
Accuracy Score | >98% | Add retrieval-augmented generation context |
If your results fall short, adjust your prompts to better align with your goals:
"Their 214-test evaluation suite reduced hallucination rates from 8.2% to 1.4% over three months while maintaining 98.7% factual accuracy."
– Shopify Engineering Team, April 2024
To maintain consistent performance, enable automatic evaluation triggers with trigger_on_model_update: true
. The next section will share tips for refining your evaluations even further.
Tips for Better Evaluations
Choosing Test Data
Using high-quality test data is key to reliable evaluations. Your test datasets should reflect real-world scenarios. For instance, if you're working on a customer service chatbot, here's a recommended data mix:
Data Type | Suggested Distribution | Example Use Cases |
---|---|---|
Common Cases | 60% | Order tracking, account queries |
Complex Scenarios | 30% | Multi-step transactions |
Edge Cases | 10% | Multi-language inputs, extreme values |
It's better to rely on real user interactions instead of synthetic data. For example, a fintech company improved evaluation accuracy by 34% by using actual customer logs, maintaining a 3:1 ratio of positive-to-negative examples. Additionally, set up a regular testing schedule to ensure ongoing reliability.
Setting Up Regular Tests
Once you've run initial tests, automate evaluations to keep validating performance over time. A technical lead from a major retail company shared that using an 80/20 split between automated evaluations and manual reviews reduced assessment time by 60% while maintaining 92% accuracy in quality checks.
When to Trigger Automatic Tests:
- After model version updates
- During weekly regression tests
- Before deployment
Key Metrics to Monitor:
- Pass rates: Aim for over 95%
- Response latency: Keep it under 2 seconds
- Accuracy scores: Target at least 98%
- User feedback trends: Look for recurring issues
Updating Test Standards
Your evaluation criteria should evolve alongside your LLM application. Update your standards in situations like these:
- When pass rates fall by more than 15%
- If user feedback highlights new issues
- When the model's capabilities grow
- If business needs shift
For example, a healthcare tech company updated its evaluation criteria in early 2025:
Metric | Original Target | Updated Target | Reason for Update |
---|---|---|---|
Medical Accuracy | 95% | 98% | New CDC guidelines |
Response Time | <2s | <1.5s | Performance improvements |
Compliance Score | Basic | HIPAA-verified | New regulatory requirements |
Document these updates and track their impact. Companies following this approach reported a 78% improvement in catching potential issues before they reached production.
To streamline monitoring, connect your Evals API with tools like Datadog or LangSmith. This setup allows for real-time alerts and better performance tracking across your evaluation processes.
sbb-itb-7a6b5a0
Intro to LLM Evaluation w/ OpenAI Evals [Walk-Thru]
Using Evals API with OpenAssistantGPT
Integrating OpenAssistantGPT into your evaluation process allows for focused testing and ongoing performance tracking.
Testing Chatbot Output
This setup ensures chatbot responses align with accuracy, compliance, and brand guidelines.
Quality Dimension | Evaluation Method | Target Threshold |
---|---|---|
Response Accuracy | Model-graded comparison | ≥90% match |
Brand Voice Consistency | Semantic similarity | ≥0.85 score |
Compliance Rate | Pattern matching | 100% adherence |
Here’s an example from a financial services company that achieved complete regulatory compliance using this configuration:
{
"business_rules": [
{
"rule": "disclosure_required",
"condition": "mentions 'loan' or 'interest rate'",
"required_text": "Member FDIC"
}
],
"failure_action": "auto_correct"
}
Checking Prompt Performance
Beyond validating output, it’s crucial to regularly assess prompts to ensure they remain effective. Automate weekly evaluations to measure response coherence, user satisfaction, and processing time. For instance, if coherence scores drop by 15% compared to the previous week, the system can trigger an alert for review.
To compare performance across different prompt versions, use the compare_runs
endpoint:
results = openai.Eval.compare(
run_ids=["prompt_v1_0324", "prompt_v2_0425"],
metrics=["conversion_rate", "avg_rating"]
)
Meeting Business Standards
Aligning chatbot outputs with business requirements is equally important. Use layered compliance checks to maintain quality across various scenarios. For example, in customer support or lead generation workflows, create templates to ensure consistent responses. In healthcare applications, strict accuracy standards are a must - many organizations require 99.8% accuracy for medical information.
Automate validation processes to verify adherence to industry regulations, ensure brand consistency, and monitor response appropriateness. For example, a retail chatbot reduced early cancellations by 42% by implementing pre-response validation, requiring agents to offer multiple troubleshooting options before processing cancellations.
For enterprise-level deployments, separate evaluation suites can help maintain high standards:
- Legal Compliance: 100% pass rate
- User Experience: ≥90%
- Brand Alignment: ≥0.85 similarity
This multi-tiered approach ensures outputs meet business expectations and maintain consistent quality.
Conclusion
The OpenAI Evals API transforms chatbot testing into a structured quality assurance process. Enterprise teams have reported 40% faster iteration cycles and $150,000 in annual QA savings.
"By implementing syntax checks, semantic validation, and contextual safety testing, organizations can achieve comprehensive quality control while streamlining their development process."
This system ensures thorough quality control while simplifying workflows. With seamless CI/CD integration, evaluations are automated after every commit, helping maintain consistent quality standards. Early adopters have seen a 34% improvement in response accuracy.
For OpenAssistantGPT developers, the API offers a dependable framework to enhance chatbot performance. For example, HealthTech Inc. reduced support ticket escalations by 30% through weekly evaluation cycles.
Looking ahead, new features like multi-turn conversation testing and automatic adversarial test generation are set to launch in Q2 and Q3 2025. These updates will further enhance the platform's ability to deliver consistent, high-quality chatbot performance across various applications.
FAQs
What are the advantages of using the OpenAI Evals API for businesses that rely on language models?
The OpenAI Evals API helps businesses ensure their language model applications meet specific style and content standards by allowing them to test and evaluate outputs programmatically. This is especially useful when upgrading models or introducing new ones, as it ensures consistent performance and reliability.
By using the Evals API, businesses can:
- Define clear evaluation criteria for their language models.
- Test outputs against real-world scenarios to identify areas for improvement.
- Iterate and refine prompts to optimize model performance over time.
This process not only improves application reliability but also saves time and resources by streamlining the evaluation workflow.
How can I keep my API key secure when using the OpenAI Evals API?
To ensure the security of your API key when using the OpenAI Evals API, follow these best practices:
- Keep your API key private: Never share your key publicly, including in code repositories, forums, or documentation.
- Use environment variables: Store your API key in environment variables instead of hardcoding it into your application.
- Regenerate compromised keys: If you suspect your API key has been exposed, immediately revoke it and generate a new one.
- Limit access: Use role-based access control and permissions to restrict who can view or use your key.
By taking these precautions, you can minimize the risk of unauthorized access to your API key and maintain the security of your application.
How can I set up and update evaluation criteria using the OpenAI Evals API?
To set up and update evaluation criteria with the OpenAI Evals API, follow these best practices:
- Define your evaluation goals: Clearly specify the style and content requirements you want your model to meet. This ensures your evaluations are aligned with your application's objectives.
- Test with representative inputs: Use realistic prompts and data that reflect how your application will be used. This helps you identify potential issues early.
- Iterate and refine: Analyze the results of your evaluations, adjust your prompts or criteria as needed, and re-run tests to improve performance over time.
By regularly updating your evaluation criteria, you can ensure your application stays reliable, especially when upgrading or experimenting with new models.